Integration Testing REST API with Spring Boot Part 4
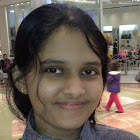
Integration Tests For Delete
@Test
@SqlGroup({
@Sql(executionPhase = ExecutionPhase.BEFORE_TEST_METHOD, statements = "insert into `comment` (id, post_id, message) values (1, 1, 'testCommentFor delete');"),
@Sql(executionPhase = ExecutionPhase.AFTER_TEST_METHOD, statements = "delete from comment;") })
public void deleteComment_forValidRequest_returnsResponseCode204() throws Exception {
mvc.perform(delete("/comments/1")).andExpect(status().isNoContent()).andDo(print());
}
@Test
@SqlGroup({
@Sql(executionPhase = ExecutionPhase.BEFORE_TEST_METHOD, statements = "insert into `comment` (id, post_id, message) values (1, 1, 'testCommentFor delete');"),
@Sql(executionPhase = ExecutionPhase.AFTER_TEST_METHOD, statements = "delete from comment;") })
public void deleteComment_forValidRequest_deletesComment() throws Exception {
mvc.perform(get("/posts/1/comments"))
.andExpect(jsonPath("$.length()", is(1)))
.andExpect(jsonPath("$.[0].id", is(1)))
.andDo(print());
mvc.perform(delete("/comments/1")).andDo(print());
mvc.perform(get("/posts/1/comments"))
.andExpect(jsonPath("$.length()", is(0)))
.andDo(print());
}
@Test
@Sql(executionPhase = ExecutionPhase.BEFORE_TEST_METHOD, statements = "delete from comment;")
public void deleteComment_whenCommentDoesNotExist_returnsResponseCode404() throws Exception {
mvc.perform(delete("/comments/1")).andExpect(status().isNotFound()).andDo(print());
}
Please find the complete source code @ https://github.com/JavaCodeHouse/comments-application