What is Spring Boot
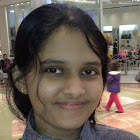
If you’ve ever set up a spring application in the past, you would know the pain!
How much configuration that has to be done.
Well, Spring Boot is here to make the developer’s life easier.
Spring Boot still uses the Spring framework under the hood, but almost all of the configuration is done for you if you wish.
Whether you use Spring Boot or Spring framework, you would still create a Spring application at the end. The differences are in the process. How fast and how easy things can get during development.
So in this post let’s take a look at the Spring Boot framework.
What is Spring Boot?
Spring Boot is a framework which helps create standalone Spring applications that are production-grade. It’s built on top of the Spring framework and takes an opinionated view of the Spring platform to minimize the configuration required by a typical Spring application. This makes it fast and easy to get started with.
Spring Boot is a framework or rather a tool built on top of spring framework which enables you to quickly bootstrap a Spring application from scratch.
So it’s more like you take a short cut to create a Spring application.
In other words, Spring Boot is an extension of the Spring framework, intended to simplify and accelerate the development of enterprise applications.
You can set up a spring application using Spring Boot (without having to write a single line of XML or any configuration what so ever) to the point where it is ready to be deployed and run in a matter of minutes . So it spares the developers more time to focus on implementing the application logic rather than project set up and configuration.
How is it possible?
Well, Spring Boot starters and Autoconfiguration makes the project set up so quick and easy that you only have to know which kind of application you want to create.
For example, if you tell the Spring Initializr that you want to create a web application, it will create the build file with all the required dependencies and the project folder structure along with a sample application class which you can just run.
But this doesn’t mean that you are confined to what has been chosen or configured by the framework for you. You can customize whatever is needed according to your preference or requirement.
So starters and auto-configuration speed up the project set up and eliminate configuration but what makes it production-ready?
It’s the Spring Boot Actuator, which lets you monitor and interact with the application once it’s deployed. The Actuator can also be configured to support CORS.
Oh, by the way, Spring Boot also embeds a server, such as TOMCAT or Reactor Netty depending on the kind of application that you create, so You can bundle up your web application as a jar file and just run it without having to have a server.
Spring Boot Starters
Often times dependencies could be tricky, there are so many external libraries and selecting versions that should be compatible with each other can be difficult at times.
But there’s a one-stop-shop for all the dependency requirements of a Spring Boot project.
That’s the spring boot starters.
All you have to do is select a few starter dependencies according to the type of project and the starter dependencies will take care of the rest.
So if you are building a REST API or a web project, you would add the spring-boot-starter-web
dependency to your project and if you take a look its build.gradle
file you can see all the other dependencies it includes.
plugins {
id "org.springframework.boot.starter"
}
description = "Starter for building web, including RESTful, applications using Spring MVC. Uses Tomcat as the default embedded container"
dependencies {
api(project(":spring-boot-project:spring-boot-starters:spring-boot-starter"))
api(project(":spring-boot-project:spring-boot-starters:spring-boot-starter-json"))
api(project(":spring-boot-project:spring-boot-starters:spring-boot-starter-tomcat"))
api("org.springframework:spring-web")
api("org.springframework:spring-webmvc")
}
Similarly, if you want to use Spring Data Jpa, you would add the spring-boot-starter-data-jpa
dependency to your project.
There’s a list of all the starters available in the Spring Boot Documentation along with a brief description of each, and the table below is derived from it.
List of popular Spring Boot starter dependencies, each with the link to its build.gradle
file
Name | Description |
---|---|
spring-boot-starter | Core starter, including auto-configuration support, logging and YAML |
spring-boot-starter-activemq | Starter for JMS messaging using Apache ActiveMQ |
spring-boot-starter-amqp | Starter for using Spring AMQP and Rabbit MQ |
spring-boot-starter-aop | Starter for aspect-oriented programming with Spring AOP and AspectJ |
spring-boot-starter-artemis | Starter for JMS messaging using Apache Artemis |
spring-boot-starter-batch | Starter for using Spring Batch |
spring-boot-starter-cache | Starter for using Spring Framework’s caching support |
spring-boot-starter-data-cassandra | Starter for using Cassandra distributed database and Spring Data Cassandra |
spring-boot-starter-data-cassandra-reactive | Starter for using Cassandra distributed database and Spring Data Cassandra Reactive |
spring-boot-starter-data-couchbase | Starter for using Couchbase document-oriented database and Spring Data Couchbase |
spring-boot-starter-data-couchbase-reactive | Starter for using Couchbase document-oriented database and Spring Data Couchbase Reactive |
spring-boot-starter-data-elasticsearch | Starter for using Elasticsearch search and analytics engine and Spring Data Elasticsearch |
spring-boot-starter-data-jdbc | Starter for using Spring Data JDBC |
spring-boot-starter-data-jpa | Starter for using Spring Data JPA with Hibernate |
spring-boot-starter-data-ldap | Starter for using Spring Data LDAP |
spring-boot-starter-data-mongodb | Starter for using MongoDB document-oriented database and Spring Data MongoDB |
spring-boot-starter-data-mongodb-reactive | Starter for using MongoDB document-oriented database and Spring Data MongoDB Reactive |
spring-boot-starter-data-neo4j | Starter for using Neo4j graph database and Spring Data Neo4j |
spring-boot-starter-data-r2dbc | Starter for using Spring Data R2DBC |
spring-boot-starter-data-redis | Starter for using Redis key-value data store with Spring Data Redis and the Lettuce client |
spring-boot-starter-data-redis-reactive | Starter for using Redis key-value data store with Spring Data Redis reactive and the Lettuce client |
spring-boot-starter-data-rest | Starter for exposing Spring Data repositories over REST using Spring Data REST |
spring-boot-starter-data-solr | Starter for using the Apache Solr search platform with Spring Data Solr |
spring-boot-starter-freemarker | Starter for building MVC web applications using FreeMarker views |
spring-boot-starter-groovy-templates | Starter for building MVC web applications using Groovy Templates views |
spring-boot-starter-hateoas | Starter for building hypermedia-based RESTful web application with Spring MVC and Spring HATEOAS |
spring-boot-starter-integration | Starter for using Spring Integration |
spring-boot-starter-jdbc | Starter for using JDBC with the HikariCP connection pool |
spring-boot-starter-jersey | Starter for building RESTful web applications using JAX-RS and Jersey. An alternative to spring-boot-starter-web |
spring-boot-starter-jooq | Starter for using jOOQ to access SQL databases. An alternative to spring-boot-starter-data-jpa or spring-boot-starter-jdbc |
spring-boot-starter-json | Starter for reading and writing json |
spring-boot-starter-jta-atomikos | Starter for JTA transactions using Atomikos |
spring-boot-starter-jta-bitronix | Starter for JTA transactions using Bitronix. Deprecated since 2.3.0 |
spring-boot-starter-mail | Starter for using Java Mail and Spring Framework’s email sending support |
spring-boot-starter-mustache | Starter for building web applications using Mustache views |
spring-boot-starter-oauth2-client | Starter for using Spring Security’s OAuth2/OpenID Connect client features |
spring-boot-starter-oauth2-resource-server | Starter for using Spring Security’s OAuth2 resource server features |
spring-boot-starter-quartz | Starter for using the Quartz scheduler |
spring-boot-starter-rsocket | Starter for building RSocket clients and servers |
spring-boot-starter-security | Starter for using Spring Security |
spring-boot-starter-test | Starter for testing Spring Boot applications with libraries including JUnit, Hamcrest and Mockito |
spring-boot-starter-thymeleaf | Starter for building MVC web applications using Thymeleaf views |
spring-boot-starter-validation | Starter for using Java Bean Validation with Hibernate Validator |
spring-boot-starter-web | Starter for building web, including RESTful, applications using Spring MVC. Uses Tomcat as the default embedded container |
spring-boot-starter-web-services | Starter for using Spring Web Services |
spring-boot-starter-webflux | Starter for building WebFlux applications using Spring Framework’s Reactive Web support |
spring-boot-starter-websocket | Starter for building WebSocket applications using Spring Framework’s WebSocket support |
What is Autoconfiguration
Autoconfiguration is the configuration of the Spring Application Context by the Spring Boot framework, attempting to guess and configure beans that you are likely to need.
If you have worked with a typical spring application, you should be familiar with all the configurations that are needed such as, data sources, entity manager factories, dispatcher servlets and the list goes on.
How autoconfiguration works
The spring boot framework kind of looks at the jar dependencies that you have added and the existing configurations for the application if there is any, and then based on these, automatically configures your Spring application.
For example, if H2 is on your classpath, and you have not manually configured any database connection beans, then Spring Boot auto-configures an in-memory database. How convenient is that?
However, auto-configuration is non-invasive. you can define your own configuration to replace specific parts of the auto-configuration as and when you want, but initially it is so much faster and easier to get started without having to deal with a lot of configuration manually.
Auto-configuration is the reason why the Pivotal team says “It’s a Kind of Magic!”
Spring Initializr
Spring initializr is a website or a web-based tool that can be used to set up a Spring Boot project.
Of course, you can set up a Spring Boot project without using the Spring initializr, but the advantage of using the Spring initializr is that it speeds up the process and does most of the groundwork for you.
All you have to do is to go to start.spring.io and add the spring boot starter dependencies that you want, (eg: web, JPA and H2 etc…) and generate the project!
Spring initialzr will do the following:
- Creates the build file with all the required dependencies, plugins and also takes care of the versions.
- Creates the project structure or the folder structure for your project.
Now you can start implementing, and yes you can do any customizations to the build file or the project setup as much as you like.
Want To Get Started With Spring Boot?
Check out the FREE course.