REST API with Spring Boot | Lesson 11 | POST Request Testing And Implementation
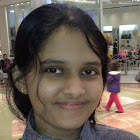
POST Request
Controller
@PostMapping("posts/{id}/comments")
public Comment addComment(@RequestBody Comment comment, @PathVariable("id") int postId) {
validateComment(comment, postId);
comment.setPostId(postId);
return commentService.createComment(comment);
}
private void validateComment(Comment comment, int postId) {
if (postId < 1) {
throw new InvalidParameterException();
}
if (comment.getId() > 0) {
throw new InvalidParameterException();
}
if (comment.getMessage() == null || comment.getMessage().isEmpty()) {
throw new InvalidParameterException();
}
}
Service
public Comment createComment(Comment comment) {
if(comment.getPostId()<1) {
throw new InvalidParameterException();
}
return commentRepository.save(comment);
}
Please find the complete source code @ https://github.com/JavaCodeHouse/comments-application