Java Optional | How and When to use it
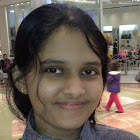
In Java 8, the Optional
class was introduced to address the problem of handling potentially null values in a more expressive and safer way.
Here are some key aspects of using Optional
, along with best practices.
How to use Optional
How to create an Optional
-
Use
Optional.of(value)
when you are certain that the value is non-null. -
Use
Optional.ofNullable(value)
when the value might be null.
String nonNullValue = "Hello";
Optional String optional1 = Optional.of(nonNullValue);
String nullableValue = null;
Optional<String> optional2 = Optional.ofNullable(nullableValue);
How to access the value of an Optional
-
Use
get()
cautiously; it throwsNoSuchElementException
if the value is not present. -
Prefer
orElse(defaultValue)
ororElseGet(() -> defaultValue)
to provide a default value when the original value is not present.
Optional<String> optional = // ...
// Avoid using get() without checking isPresent()
String value = optional.orElse("Default");
How to avoid null checks with optional
Use ifPresent(Consumer<? super T> consumer)
to perform an action only when the value is present, which eliminates the need for explicit null checks.
Optional<String> optional = // ...
optional.ifPresent(val -> System.out.println("Value: " + val));
Best Practices
Avoid using Optional for method parameters
While using Optional for method return types is acceptable, it’s generally not recommended for method parameters. It can make the code less readable and complicate the method signature.
Use Optional in Stream API
Optional
integrates well with the Stream API. For example, you can use Optional
in combination with filter
, map
, and other stream operations.
List<String> strings = // ...
Optional<String> result = strings.stream()
.filter(s -> s.startsWith("A"))
.findFirst();
Consider alternative approaches
In some cases, using Optional
might be unnecessary, and a simple null check or default value assignment could be more readable and straightforward.
When to use and not to use Optional
Use Optional
when:
- You want to make it explicit that a value might be absent.
- You want to take advantage of the functional programming features in the Stream API.
- You want to enforce explicit handling of absent values.
Avoid Optional
when:
- Dealing with method parameters; it’s generally not recommended.
- It doesn’t improve the code readability or when simpler alternatives suffice.
Optional
is a powerful tool for expressing optional values more clearly and avoiding null pointer exceptions.
However, it should be used judiciously, and developers should be mindful of its appropriate application to avoid unnecessary complexity in the code.
References
Tired of Null Pointer Exceptions? Consider Using Java SE 8’s Optional!