Java 8 Optional - Avoid the explicit null check on list
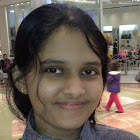
Avoid the ugly null check with Optional
How to get the first item of a nullable list in Java using Optional
Without Optional
(the traditional way)
public static void main(String[] args) {
// List<String> items = null;
List<String> items = List.of("1", "2", "3");
if (items != null && !items.isEmpty()) {
String firstItem = items.get(0);
System.out.println("First item: " + firstItem);
} else {
System.out.println("List is null or empty");
}
}
This is simple and widely understood by Java developers. May be more familiar to developers who are not yet accustomed to Java 8 features.
But it’s a bit verbose.
The null check and the access to the first element are combined in a single block, potentially making the code less expressive.
With Optional (More expressive way)
public static void main(String[] args) {
// List<String> items = null;
List<String> items = List.of("1", "2", "3");
Optional.ofNullable(items)
.flatMap(list -> list.stream().findFirst())
.ifPresentOrElse(
item -> System.out.println("First item: " + item),
() -> System.out.println("List is null or empty")
);
}
}
Using map
method instead of flatMap
(still using Optional)
public static void main(String[] args) {
// List<String> items = null;
List<String> items = List.of("1", "2", "3");
Optional.ofNullable(items)
.map(list -> list.stream().findFirst())
.ifPresentOrElse(
item -> System.out.println("First item: " + item.get()),
() -> System.out.println("List is null or empty")
);
}
Using Optional expresses the intent more clearly: “I want the first item of the list if it is present and not empty.”
Separates the null check, filtering, and mapping, making each step more explicit.
Which method would you use?